Rule can be written in the form of a JavaScript function.
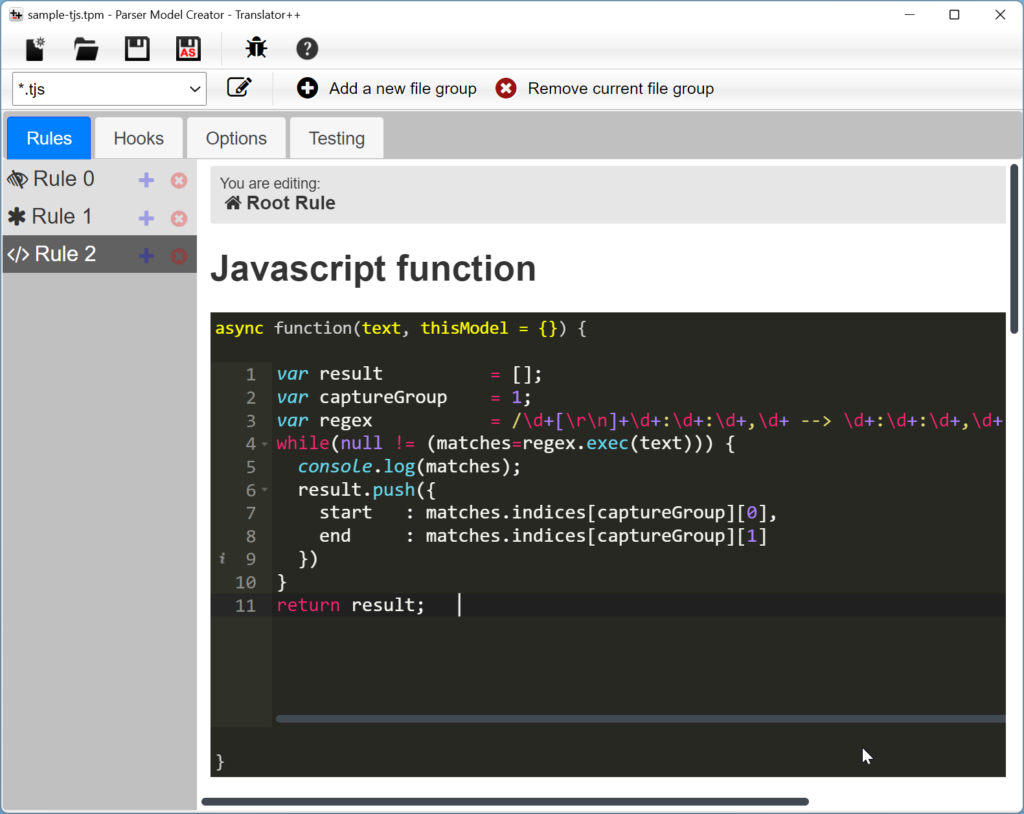
Function wrapper
Your code is going to be wrapped with the following function:
async function(text, thisModel = {}) {
// your code
}
You can access arguments
object and this
keyword.
Arguments
The code you write will be inside a function with 2 arguments that can be accessed anywhere in your code:
- textStringA string of the entire content of the file.
- thisModelObjectThe rule object that is currently used by the file.
this Keyword
this will reffer to the instance of CustomParser class
.
Expected Return Value
Your code must return one of the following value:
undefined
- Offset of the captured text, for example:
{
start:100,
end:120
}
- Array of the offset of the captured text, for example:
[
{start:0, end:5},
{start:12, end:30},
{start:4581, end:4593}
]
Example
The following code is an example to parse srt subtitle.
/*
Translator++ is using regexp-match-indices
by that all RegExp.exec() method will return their group offset
read more about Regular Expression Indices here: https://v8.dev/features/regexp-match-indices
*/
var result = [];
var captureGroup = 1;
var regex = /\d+[\r\n]+\d+:\d+:\d+,\d+ --> \d+:\d+:\d+,\d+[\r\n]+((.+\r?\n)+(?=(\r?\n)?))/g;
while(null != (matches=regex.exec(text))) {
console.log(matches);
result.push({
start : matches.indices[captureGroup][0],
end : matches.indices[captureGroup][1]
})
}
return result;